01. Register in kookoo
TestLink : http://www.kookoo.in
Register your account from above link, and fill all the details.02. Get your authentication details
Login with your username and password, and Go to http://www.kookoo.in/index.php/dashboardv2 page. Get your API Key, this credentials will required in your REST API.03. Text to Speech API
Now using IndianTTS Text to Speech API and convert your text to audio format, refer bellow screen shot for TEXT to Speech API<?php header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Credentials: true "); header("Access-Control-Allow-Methods: OPTIONS, GET, POST"); header("Access-Control-Allow-Headers: Content-Type, Depth, User-Agent, X-File-Size, X-Requested-With, If-Modified-Since, X-File-Name, Cache-Control"); $host = '<TTS API HOST>'; $port = '<PORT>'; $text = <TEXT>; $url = "http://".$host.":".$port."/tts?text=".$text; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_PORT => "<PORT>", CURLOPT_URL => $url, CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; } ?>
04. Integrate kookoo API code
Test Audio URL (which is return from TTS) integrate with kookoo API code, code screen shot is bellow<?php header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Credentials: true "); header("Access-Control-Allow-Methods: OPTIONS, GET, POST"); header("Access-Control-Allow-Headers: Content-Type, Depth, User-Agent, X-File-Size, X-Requested-With, If-Modified-Since, X-File-Name, Cache-Control"); $host = 'www.kookoo.in'; $audio = $_POST['audio']; $mobile = $_POST['mobile']; $url = "http://".$host."/outbound/outbound.php?phone_no=". $mobile ."&api_key=<APIKEY>&outbound_version=2&extra_data=<response><playaudio>".$audio."</playaudio></response>"; $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => $url, CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; } ?>
05. Log Entry Provide by KooKoo
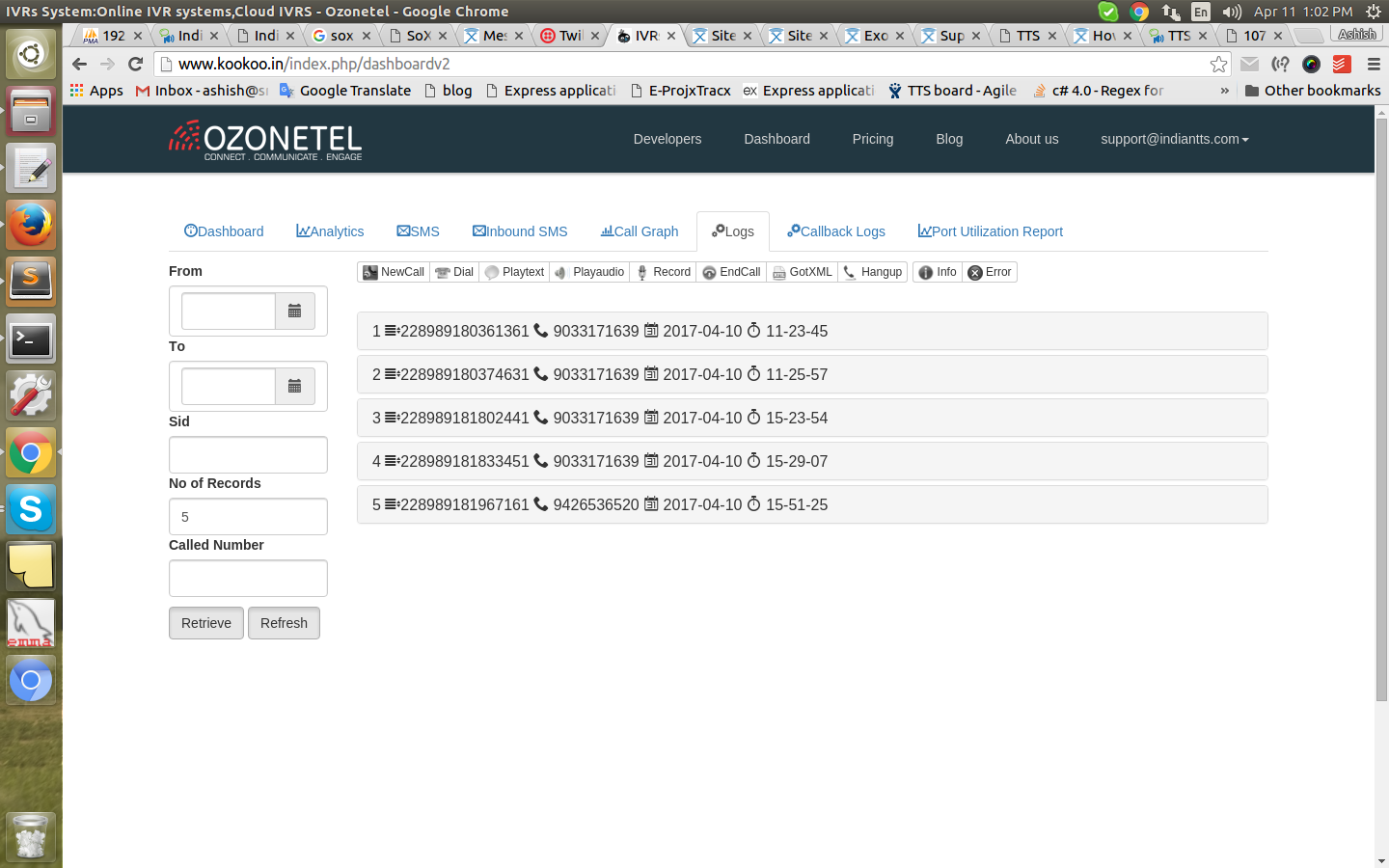